How to Add a Custom Email Token to Mautic
In today’s post, we’ll explore how to make Mautic recognize and use a custom email token. By the end of this tutorial, you’ll have a working example of a custom token that formats an IBAN (International Bank Account Number) for use in your emails.
If you’re eager to see the final result, check out the branch from this repository:
Prerequisites
Before starting, ensure you’ve installed Mautic and an empty “Hello World” bundle as shown in the previous tutorial.
What Token Are We Adding?
We’re going to:
Add an iban custom field to the database.
Register a token called {customiban} that can be used in email templates. This token will format and display the IBAN properly when leads receive the email.
Step 1: Create the IBAN Field
Start by adding a custom field named iban in Mautic. Assign it to the core field group. This ensures the field is available for all leads in your Mautic instance.
Step 2: Hook into the Mautic Lifecycle
To make Mautic recognize our token, we need to hook into the right event. Below is the class that does exactly that:
<?php
declare(strict_types=1);
namespace MauticPlugin\HelloWorldBundle\EventListener;
use Mautic\CoreBundle\Event\TokenReplacementEvent;
use Mautic\EmailBundle\EmailEvents;
use Mautic\LeadBundle\Entity\Lead;
use Mautic\LeadBundle\Model\LeadModel;
use Psr\Log\LoggerInterface;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
class EmailSubscriber implements EventSubscriberInterface
{
public const IBAN_TOKEN = '{customiban}';
public function __construct(
private LeadModel $leadModel,
private LoggerInterface $logger
) {
}
public static function getSubscribedEvents(): array
{
return [
EmailEvents::TOKEN_REPLACEMENT => [
['onEmailTokenReplacement', 0],
],
];
}
private function extractIbanFromLead($lead): string
{
$iban = '';
if (is_array($lead) && isset($lead['iban'])) {
$iban = $lead['iban'];
} elseif ($lead instanceof Lead) {
$iban = $lead->getFieldValue('iban', 'core');
}
return $iban;
}
private function formatIban(string $iban): string
{
$cleanedIban = str_replace(' ', '', $iban);
return preg_replace(
'/(\w{4})(\w{4})(\w{4})(\w{4})(\w+)/', '$1 $2 $3 $4 $5',
$cleanedIban
);
}
public function onEmailTokenReplacement(TokenReplacementEvent $event): void
{
$event->addToken(self::IBAN_TOKEN, '');
$lead = $event->getLead();
$formattedIban = $this->formatIban(
$this->extractIbanFromLead($lead)
);
$event->addToken(self::IBAN_TOKEN, $formattedIban);
}
}
Code Breakdown
Declaring the Custom Token: We define {customiban} as our custom token.
Extracting the IBAN:
The extractIbanFromLead method retrieves the IBAN value from the lead data.
It handles both associative arrays and Lead objects for compatibility.
Formatting the IBAN:
The formatIban method cleans the IBAN string and formats it for readability (e.g., splitting it into blocks of four).
Replacing the Token:
In onEmailTokenReplacement, we:
Set a default empty value for the token to ensure graceful fallback.
Use the extracted and formatted IBAN to replace the token in the email.
Step 3: Test Your Token
Now that Mautic knows about the {customiban} token, you can use it in an email. Create a new email template, insert the {customiban} token, and send it to a lead with an IBAN value. The IBAN will appear nicely formatted in the email.
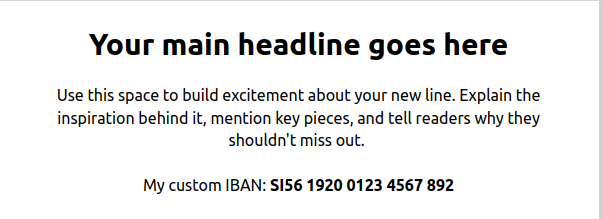
Conclusion
This tutorial demonstrated how to register and use a custom email token in Mautic. While we focused on email tokens, the same principles apply to other contexts like SMS tokens. For example, you can subscribe to the Mautic\SmsBundle\SmsEvents::TOKEN_REPLACEMENT event to add SMS-specific tokens.
Possible Improvements
Token autocomplete in the email editor.
More robust formatting options for IBANs in different regions.
These topics are beyond the scope of this tutorial but provide exciting opportunities for further development.